Using Request & Response
In this section we will test the request & response mechanism by making a webpage with an HTML Form that is used to send the paramaters to a Python script that stores and shows the form's input:
Create an HTML file from listing 2, and test it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<!DOCTYPE html> <html><head><meta charset="UTF-8"></head> <body> <form action="formreceiver.py" method="get"> What is your name?<br> <input type="text" name="name"><br> What is the sport you play?<br> <select name="sport"> <option>basketball <option>volleyball <option>soccer <option>handball <option>other or none </select><br> What year did you start playing this sport?<br> <input type="text" name="year"><br> <input type=submit name="submit" value="submit"> </form> </body> </html> |
Observe and try to understand the following:
- The
action="formreceiver.py"
parameter in line4 tells the browser to collect the forms' content in aRequest
object and send that to be dealt with by theformrecevier.py
script. - This currently results in an error, because the script you point to was not created yet...
- Because you specify
method="get"
to be used, the parameters have been added to the URL (as Key-Value pairs).
To get at Request
object data in your Python script, you use the cgi.FieldStorage
class. This reads the form contents, either from the QueryString
URL or from the hidden FORM
object.
The FieldStorage
instance can be indexed like a Python dictionary. It allows membership testing with the in
operator, and also supports the standard dictionary method keys()
and the built-in function len()
. Any fields containing empty strings are ignored and do not appear in the dictionary.
If the submitted form data contains more than one field with the same name, the object retrieved by cgi.FieldStorage
is not a FieldStorage instance but a list
of such instances. Similarly, in this situation, cgi.FieldStorage.getvalue(key)
would return a list of strings. This can get confusing quickly, and therefore the interface provides two high-level convenience methods:
FieldStorage.getfirst(name, default=None)
- This method always returns only one value associated with form field name. The method returns only the first value in case that more values were posted under such name. Please note that the order in which the values are received may vary from browser to browser and should not be counted on. If no such form field or value exists then the method returns the value specified by the optional parameter
FieldStorage.getlist(name)
default. This parameter defaults to “None” if not specified. FieldStorage.getlist(name)
- This method always returns a list of values associated with form field name. The method returns an empty list if no such form field or value exists for name. It returns a list consisting of one item if only one such value exists.
To receive input you will now create a CGI script called formreceiver.py
. It should contain code from listing 3 that reads the contents of the Request
object sent by the form.html
file you made earlier.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#import cgi module: import cgi #output http header: print ('Content-type: text/html') print ('') # note the empty print above is required! print ('<html><head><title>Python Test</title></head>') print ('<body>') theRequest = cgi.FieldStorage() theName = theRequest.getfirst("name", "undefined") print ('</body></html>') |
Note that when you test now, you will will get no error messages, but also nothing really seems to happen, leaving you with a blank web page... You do retrieve the data of the Request
object into a variable called theRequest
in line 12. Line 13 then puts the content of the name
form field into a variable theName
. But none of this is noticable, because you do NOT report anything to the user yet. You are going to do that in the next task, employing the Response
object.
You now should use this method to make the CGI formreceiver.py
not only retrieve the data, but also process it and then ‘echo’ the results back to the user, as shown in the figure below:
- The name of the user who filled in the form;
- His or her favorite sport;
- The amount of years he or she has been playing this sport. For this you’ll need to process the input!
You will have to figure out yourself how to achieve the goals listed above. A possible solution is listed below (available only at certain dates) but please try to figure things out for yourself first! You can use the Python knowledge you already have, plus the references to relevant modules help on the next page...
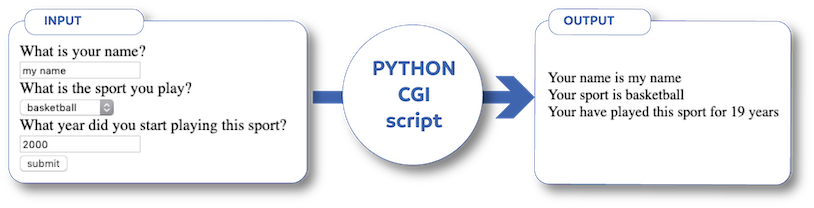