HTML and Javascript
Dynamic HTML and scripting
Dynamic HTML (DHTML) is normal HTML with an added twist... That is, DHTML includes all the elements that make up a traditional web page. However, with DHTML all of those elements are now programmable objects, collected in the Document Object Model (DOM) of the webpage. You can select each element of an HTML page, e.g. by assigning each element a unique ID and then use scripting to alter the elements after the page has been downloaded. In section 3 you will learn how to work with the HTML DOM.
We have created a small webpage where you will find examples of how this works and what you can achieve with scripting DHTML.
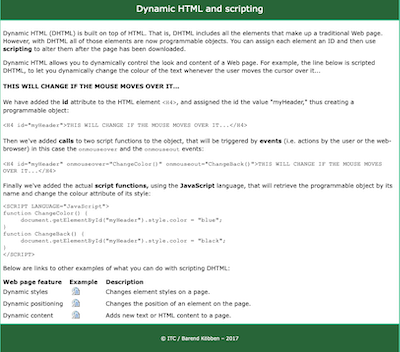
Study the examples on the webpage JavascriptExamples carefully and try to figure out how the principle works. In the rest of this exercise you will build similar things, and you can use the examples here to understand and get inspired...
The Javascript language
From the name alone, you might think Javascript is like the programming language Java. However, the Javascript language resembles Java in some ways in its syntax, but in most other ways is quite different. In comparison to other languages, such as Python, it has some similarities and some peculiarities:
It is case–sensitive (like Python):
The variable
mysomething
is different from the variable
MySomeThing
.
It is object-oriented (like Python):
There are objects, such as
document
that have methods
document.write()
which take arguments
document.write(“a string” + aVariable)
and have fields HTMLElement.visibility
, which can be read
isVisible = HTMLElement.visibility
and set
HTMLElement.visibility = true
But unlike Python, delimitation and blocking is done by using parentheses and curly braces respectively:
if (decision) then { do all these things }
within blocks, statement separation is done with semi-colons:
if (decision) then {do one thing; do another thing}
It has loose typing, because objects can be unassigned/untyped and are mutable. This means that variable data types do not have to be declared beforehand, and can change: if you assign a string
value to a variable, it automatically will become a string type variable! Consider the following code:
let A; [the type of A is undefined, and has no value] let B = 3; [the type of B is number, and the value is 3] A = “5”; [the type of A now changes to string, and the value becomes "5"] C = A + B; [the type of C is string and the value = "53" ...!
Javascript is interpreted (not compiled) by the client software (which is usually the browser). The code is integrated with, and embedded in, HTML, and the next exercise will teach you how to achieve that.